Debouncing: A Technique to Improve Web Application Performance and Save Money
Debouncing is a technique used in web development to improve the performance of applications and save costs. It is particularly useful when you have long-running operations that are invoked frequently by users. By delaying the execution of such operations until a certain period of inactivity, debouncing reduces the load on the application, resulting in faster response times and reduced costs. This article explains the concept of debouncing and provides a sample implementation using JavaScript.

Debouncing is a technique that ensures that long-running operations are not executed too often. A common example is a search operation that is triggered whenever a user types a query in an input field. Debouncing this operation would mean waiting for the user to stop typing for a particular time (usually in milliseconds) before running the search operation.
To implement debouncing, you can use a simple function that takes in another function and a time value as parameters. This function returns another function that uses a local variable, timer
, to manage the timing. Whenever the returned function is invoked, it first clears any existing timer and sets a new one to delay the execution of the original function.
Here's an example implementation of a debouncing function in JavaScript:
function debounce(func, time = 300) { let timer; return (...args) => { clearTimeout(timer); timer = setTimeout(() => func.apply(this, args), time); }; }
const nameElement = document.querySelector("#search"); function debouncedSearchFunction(event) { console.log("querying: " + event.target.value + "...."); } const debouncedSearch = debounce(debouncedSearchFunction, 500); nameElement.addEventListener("keyup", (event) => { debouncedSearch(event); });
In this example, the debouncedSearch
function is created using the debounce
function defined earlier. Whenever the user types something in the input field, the keyup
event is triggered and the debouncedSearch
function is called with the event as its argument.
By using debouncing, you can improve the performance of your web application and reduce the number of requests you make to an API endpoint, which can help you save money.
What's Your Reaction?




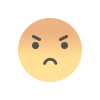

