Vue3 core Typescript class analysis
Different from using JavaScript, writing Vue programs in Typescript requires knowledge of Vue related types. Most of the Vue core types are written in the @vue/runtime-core package.
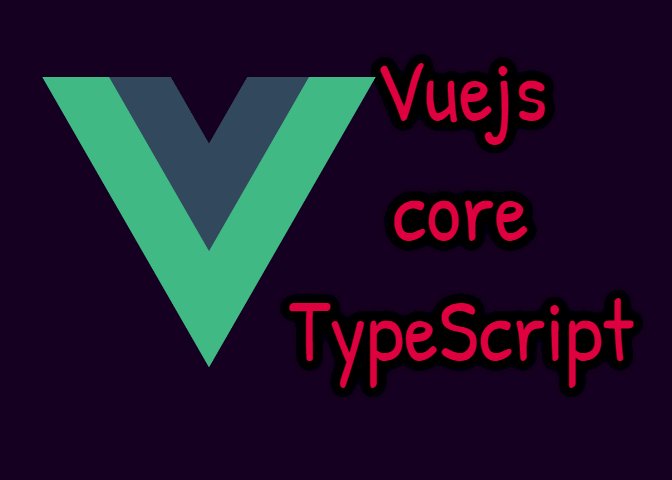
Different from using JavaScript, writing Vue programs in Typescript requires knowledge of Vue related types. Most of the Vue core types are written in the @vue/runtime-core package.
Component
The Vue page is composed of components. The classes of components in Vue are Component
inherited ComponentOptions
, FunctionalComponent
and ComponentPublicInstanceConstructor
.
Among them, ComponentOptions
inherited ComponentOptionsBase
is the option component of declarative inclusion data
and methods
other attributes that we often write :
FunctionalComponent
Is a functional component, ComponentPublicInstanceConstructor
is an instance constructor (constructor).
ComponentOptions
Inherited ComponentCustomOptions
, this interface is empty in the Vue source code. We can use it to customize the attributes in the Vue component options, such as the example in the source code:
declare module '@vue/runtime-core' {
interface ComponentCustomOptions {
beforeRouteUpdate?(
to: Route,
from: Route,
next: () => void
): void
}
}
The defineComponent
function we use when defining the component is used to help us make the type declaration of the component option, it accepts ComponentOptionsWithoutProps
, ComponentOptionsWithArrayProps
or ComponentOptionsWithObjectProps
as an option parameter. They are all inherited ComponentOptionsBase
, but have different forms of declaring props. This function can also accept the setup function.
defineComponent
Function returns a DefineComponent
class object, it is ComponentOptionsBase
and ComponentPublicInstanceConstructor
the intersection of class objects:
type DefineComponent = ComponentPublicInstanceConstructor & ComponentOptionsBase &&
CreateAppFunction
In V3, the start of a page is usually from the createApp
beginning, and its type declaration is like this:
export type CreateAppFunction = (
rootComponent: Component,
rootProps?: Data | null
) => App
It accepts a Component
sum attribute as a parameter and returns one App
.
App
App
An instance is a top-level object of Vue, through which you can set shared properties, set plug-ins, register components, set compilation options, set error handling functions, etc.
mount
The root component can be mounted to the document through the method, and an ComponentPublicInstance
object is returned .
ComponentPublicInstance
ComponentPublicInstance
Is a component instance, including $el
,'$emit'$props Component ComponentPublicInstance`.
Its type is defined as:
type ComponentPublicInstance<
P = {}, // props type extracted from props option
B = {}, // raw bindings returned from setup()
D = {}, // return from data()
C extends ComputedOptions = {},
M extends MethodOptions = {},
E extends EmitsOptions = {},
PublicProps = P,
Defaults = {},
MakeDefaultsOptional extends boolean = false,
Options = ComponentOptionsBase<any, any, any, any, any, any, any, any, any>
> = {
$: ComponentInternalInstance
$data: D
$props: MakeDefaultsOptional extends true
? Partial & Omit
: P & PublicProps
$attrs: Data
$refs: Data
$slots: Slots
$root: ComponentPublicInstance | null
$parent: ComponentPublicInstance | null
$emit: EmitFn
$el: any
$options: Options & MergedComponentOptionsOverride
$forceUpdate: () => void
$nextTick: typeof nextTick
$watch(
source: string | Function,
cb: Function,
options?: WatchOptions
): WatchStopHandle
} & P &
ShallowUnwrapRef &
UnwrapNestedRefs &
ExtractComputedReturns &
M &
ComponentCustomProperties
Among them $options
is the intersection of the ComponentOptionsBase
class object (if any, a renderer
method for functional components ) and MergedComponentOptionsOverride (hook function) when we write components .
P
, ShallowUnwrapRef
, UnwrapNestedRefs
, ExtractComputedReturns
, M
Reading the data component instance attributes and methods can help us to use the this [...] manner.
ComponentCustomProperties
It is an empty interface in the source code, and we can use it to customize the attributes on the component instance. Example:
import { Router } from 'vue-router'
declare module '@vue/runtime-core' {
interface ComponentCustomProperties {
$router: Router
}
}
$
Attributes are ComponentInternalInstance
class objects, representing internal examples of components, including some attributes provided for advanced applications, including VNode
.
What's Your Reaction?




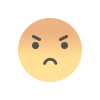

